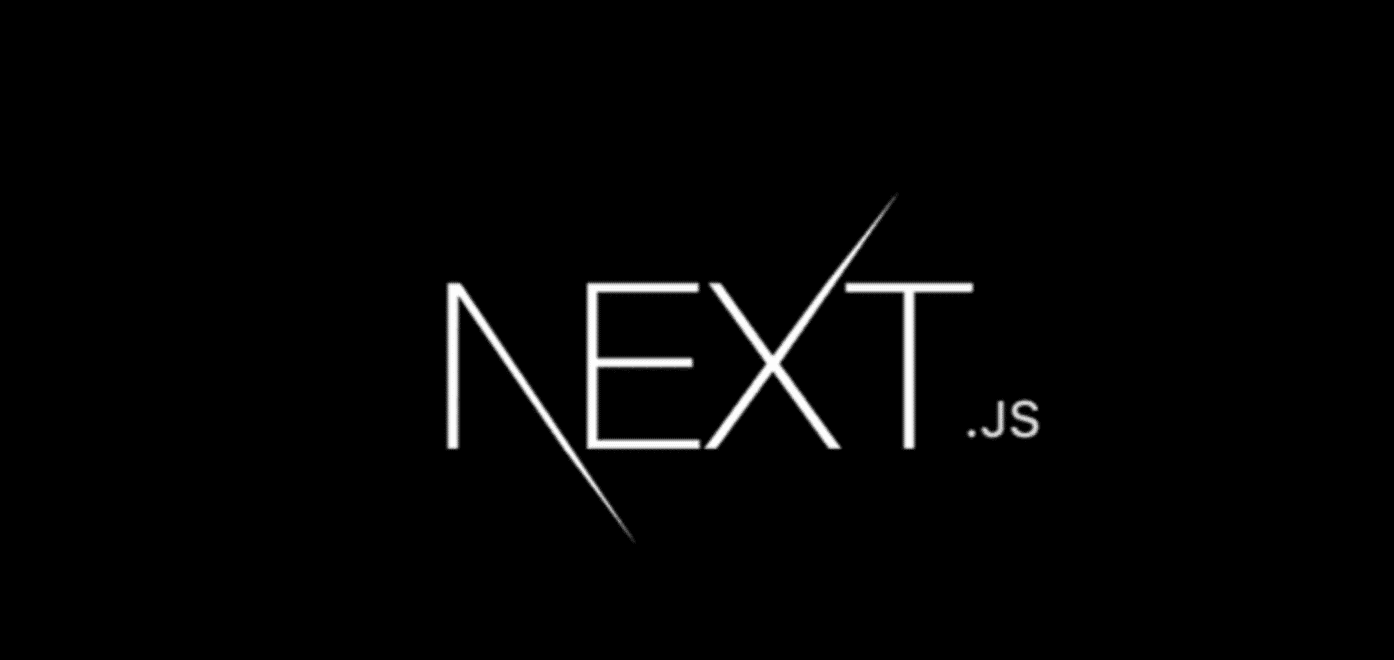
In the rapidly growing world of Next.js development, being well-prepared for interviews is essential.
This article delves into commonly asked Next.js interview questions and answers, categorized by difficulty: beginner, intermediate, and expert levels.
Are you aiming for success in your Next.js interview? Let's get you ready.
Difficulty Level: Beginner
- What is Next.js, and how does it differ from React? Next.js is an open-source framework built on React, designed for creating server-side rendered React applications. The primary distinction is in routing: React employs client-side routing, while Next.js offers server-side routing for faster loading and better SEO. It also includes features like automatic code splitting, static site generation, and dynamic imports.
- What are the advantages of using Next.js over React? Next.js offers benefits like server-side rendering, automatic code splitting, static site generation, dynamic imports, optimized performance, and easy deployment. It also supports built-in SEO and analytics, simplifying search engine optimization and user engagement tracking.
- How do you create a new Next.js application? To initiate a Next.js application, use the create-next-app command. For instance, you can run npx create-next-app my-app to create a new Next.js app called my-app.
- What is server-side rendering, and why is it important? Server-side rendering (SSR) involves rendering a webpage on the server before sending it to the client's browser. SSR is crucial for search engine indexing, improved SEO, faster initial load times, and a better experience for users on slower connections or devices.
- What is client-side rendering, and how does it differ from server-side rendering? Client-side rendering (CSR) renders a webpage on the client's browser using JavaScript after receiving initial HTML, CSS, and JavaScript from the server. SSR sends fully rendered HTML to the client, while CSR sends an empty HTML page populated by JavaScript.
- What is static site generation, and how does it differ from server-side rendering? Static site generation (SSG) creates static HTML, CSS, and JavaScript files for each page during the build process. Unlike SSR, SSG generates static files that can be served from a content delivery network (CDN).
- How do you configure routing in a Next.js application? Next.js employs file-based routing, where creating a file in the pages directory with the desired URL path automatically creates a corresponding page.
- What is the purpose of the getStaticProps function in Next.js? getStaticProps fetches data at build time for static site generation. It's called during the build process and passes the fetched data as props to the page component.
- How do you pass data between pages in a Next.js application? Next.js offers various methods to pass data between pages, such as URL query parameters, the Router API, and state management libraries like Redux or React Context. The getServerSideProps function can also be used for server-side data fetching.
- How do you deploy a Next.js application? Next.js applications can be deployed on cloud services like AWS, Google Cloud Platform, Microsoft Azure, or platforms like Vercel and Netlify. Use the next export command for SSG, or platform-specific deployment tools for server-side rendering.
Difficulty Level: Intermediate
- What is serverless architecture, and how does it relate to Next.js? Serverless architecture, managed by cloud providers, scales resources based on demand. Next.js can work with serverless architecture by deploying to platforms like AWS Lambda or Google Cloud Functions.
- What is the difference between getServerSideProps and getStaticProps functions in Next.js? getServerSideProps fetches data at runtime for server-side rendering, while getStaticProps fetches data at build time for static site generation.
- What is the purpose of the getStaticPaths function in Next.js? getStaticPaths generates dynamic paths for pages with dynamic data. This function is used to create possible values for dynamic data, which are then used to generate static files.
- How do you configure dynamic routes in a Next.js application? Dynamic routes are configured in Next.js using square brackets, e.g., [slug].js for dynamic routes like /blog/[slug].
- How does Next.js handle code splitting, and why is it important? Next.js automatically divides code into smaller chunks loaded on demand when users navigate to new pages, reducing initial load times and improving performance.
- What is the purpose of the _app.js file in Next.js? _app.js wraps the entire application, providing global styles, layout components, and context providers. It's called on every page request and adds common functionality to your application.
- How do you implement authentication in a Next.js application? Authentication can be implemented in Next.js through methods like JSON Web Tokens (JWT), OAuth, or third-party libraries like NextAuth.js. Server-side authentication can also be used with session management.
- What is the difference between a container component and a presentational component? A container component manages state and logic, while a presentational component focuses on rendering UI based on props from the container component.
- What is the purpose of the useEffect hook in React, and how does it relate to Next.js? useEffect handles side effects in functional components, such as data fetching. In Next.js, it's used for client-side data fetching via libraries like Axios or SWR.
- How do you handle errors in a Next.js application? Error handling in Next.js involves custom error pages, server-side error handling with getInitialProps, and client-side error handling with React error boundaries. Third-party tools like Sentry or Rollbar can be used for error monitoring.
Difficulty Level: Expert
- How do you implement internationalization (i18n) in a Next.js application? Next.js supports i18n via libraries like next-i18next for translating content and switching languages based on user preferences or browser settings.
- What is the purpose of the getServerSideProps function in Next.js, and how does it relate to the getInitialProps function? getServerSideProps fetches data at runtime for server-side rendering, while getInitialProps (deprecated in Next.js 9.3 and later) performs similar tasks.
- How do you implement server-side caching in a Next.js application? Server-side caching is managed through the Cache-Control header. Setting cache duration with getServerSideProps or page component's cacheControl property is key. Other options include caching libraries, CDN caching, and edge caching.
- How do you optimize the performance of a Next.js application? Performance optimization strategies include code splitting, lazy loading, image optimization, server-side caching, CDN caching, and using performance monitoring tools.
- How do you implement serverless functions in a Next.js application? Serverless functions are implemented in Next.js through API Routes. Creating a file in the pages/api directory with the desired endpoint name allows you to define serverless logic.
- How do you implement a headless CMS with Next.js? Headless CMS integration involves using third-party CMS platforms like Contentful, Strapi, or Sanity. These platforms provide APIs that can be integrated with Next.js through getStaticProps or getServerSideProps.
- How do you handle SSR for complex data models or nested data structures? Complex data models can be managed using GraphQL or REST APIs for data fetching. Libraries like swr or react-query can handle data fetching and caching.
- How do you implement A/B testing in a Next.js application? A/B testing can be implemented using third-party tools like Google Optimize or Optimizely or by using custom solutions like feature flags and conditional rendering for testing different application variations.
- How do you handle real-time updates in a Next.js application? Real-time updates can be managed through server-sent events, web sockets, or libraries like Socket.io. Additional libraries like react-use or Redux can handle real-time data updates.
- How do you implement testing and continuous integration in a Next.js application? Testing and continuous integration are achieved using frameworks like Jest or Cypress for tests and services like CircleCI or Travis CI for automation. Code quality tools like ESLint and Prettier ensure code consistency and maintainability.
Conclusion
This comprehensive article provides a quick reference for Next.js interview preparation. While these questions and answers can be a valuable guide, remember that a solid foundation in Next.js development is essential to excel in the interview.